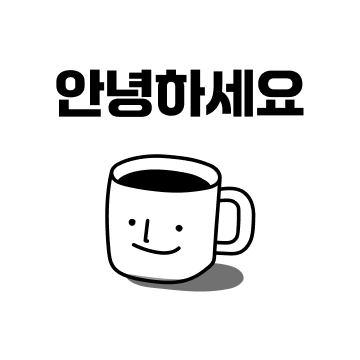
멀티스레드(Multi-threading)는 하나의 프로세스 내에서 여러 개의 스레드를 실행하는 기술을 의미합니다. 스레드는 프로세스 내에서 실행되는 가장 작은 실행 단위로, 멀티스레드를 활용하면 하나의 프로그램이 동시에 여러 작업을 수행할 수 있습니다.
1. 멀티스레드의 개념
1.1 스레드(Thread)란?
스레드는 운영체제에서 프로세스의 실행 단위를 의미하며, 하나의 프로세스는 여러 개의 스레드를 가질 수 있습니다. 모든 스레드는 같은 프로세스 내에서 메모리를 공유하며 독립적인 실행 흐름을 가집니다.
1.2 멀티스레드의 동작 방식
멀티스레드는 하나의 프로세스 내에서 여러 개의 작업을 동시에 수행하는 방식입니다. 운영체제는 스케줄러를 통해 CPU 시간을 각 스레드에 분배하며, 이를 통해 병렬 실행이 가능합니다.
2. 멀티스레드의 장점과 단점
2.1 장점
- 성능 향상: CPU를 효율적으로 사용하여 작업 처리 속도를 높일 수 있습니다.
- 자원 공유: 동일한 프로세스 내에서 메모리 공간을 공유하여 자원 사용을 최적화할 수 있습니다.
- 응답성 개선: UI 응용 프로그램에서 멀티스레드를 사용하면 사용자의 입력에 빠르게 반응할 수 있습니다.
- 동시성 지원: 여러 작업을 병렬로 실행하여 프로그램의 효율성을 높일 수 있습니다.
2.2 단점
- 동기화 문제: 여러 스레드가 같은 자원에 접근할 경우 데이터 일관성을 유지하기 어려우며 동기화 처리가 필요합니다.
- 디버깅 난이도 증가: 스레드 간 상태 변화가 복잡하여 디버깅이 어렵습니다.
- 컨텍스트 스위칭 비용: 스레드 간 전환(Context Switching) 비용이 발생하여 과도한 멀티스레드는 오히려 성능을 저하시킬 수 있습니다.
3. 멀티스레드 구현 방법
3.1 Java에서 멀티스레드 구현
자바에서는 Thread 클래스를 상속하거나 Runnable 인터페이스를 구현하여 멀티스레드를 생성할 수 있습니다.
3.1.1 Thread 클래스를 상속하는 방법
class MyThread extends Thread {
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(Thread.currentThread().getName() + " 실행 중");
}
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyThread thread2 = new MyThread();
thread1.start();
thread2.start();
}
}
3.1.2 Runnable 인터페이스를 구현하는 방법
class MyRunnable implements Runnable {
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(Thread.currentThread().getName() + " 실행 중");
}
}
}
public class RunnableExample {
public static void main(String[] args) {
Thread thread1 = new Thread(new MyRunnable());
Thread thread2 = new Thread(new MyRunnable());
thread1.start();
thread2.start();
}
}
3.2 동기화 처리 (Synchronization)
멀티스레드를 사용할 때 공유 자원에 대한 접근을 조정하기 위해 synchronized 키워드를 사용할 수 있습니다.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class SyncExample {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("최종 카운트: " + counter.getCount());
}
}
3.3 Executors를 활용한 스레드 관리
Java의 ExecutorService를 사용하면 스레드 풀을 활용하여 효율적인 스레드 관리를 할 수 있습니다.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(3);
for (int i = 0; i < 5; i++) {
executor.execute(() -> {
System.out.println(Thread.currentThread().getName() + " 실행 중");
});
}
executor.shutdown();
}
}
4. 멀티스레드를 사용할 때 주의할 점
- 데드락(Deadlock) 방지: 두 개 이상의 스레드가 서로의 자원을 점유하고 해제하지 않는 상황을 피해야 합니다.
- 레이스 컨디션(Race Condition) 해결: 여러 스레드가 동시에 자원에 접근할 때 발생하는 문제를 방지하기 위해 동기화를 고려해야 합니다.
- 적절한 스레드 개수 관리: 너무 많은 스레드를 사용하면 오히려 성능 저하를 초래할 수 있습니다.
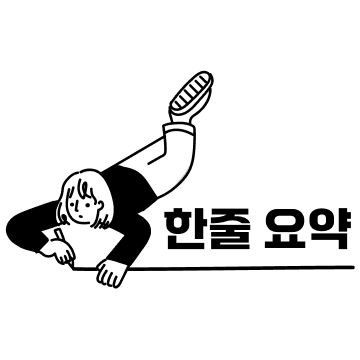
멀티스레드는 프로그램의 성능을 향상시키고 응답성을 높이지만, 동기화 문제와 스레드 관리의 복잡성을 고려해야 합니다. Java에서는 Thread 클래스, Runnable 인터페이스, 그리고 ExecutorService 등을 활용하여 구현할 수 있습니다.
'CS' 카테고리의 다른 글
API Gateway란? (0) | 2025.03.12 |
---|---|
Mock(Mocking)란 무엇인가? (1) | 2025.03.12 |
해시 테이블(Hash Table) 이란? (0) | 2025.01.23 |
CPU 스케줄링 이란? (1) | 2025.01.21 |
프로세스(Process)와 스레드(Thread) (1) | 2025.01.21 |