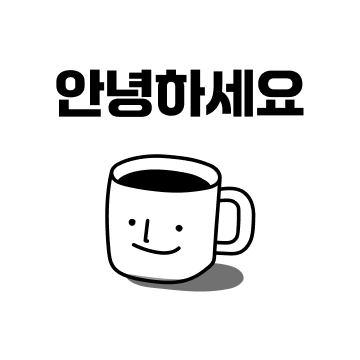
๐ง try-with-resources๋?
Java 7์์ ์ฒ์ ๋์ ๋ try-with-resources๋ ๋ฆฌ์์ค๋ฅผ ์๋์ผ๋ก ๋ซ์์ฃผ๋ ๊ตฌ๋ฌธ์ ๋๋ค. ์ด์ ์๋ finally ๋ธ๋ก์ ์ฌ์ฉํด ์ง์ ๋ฆฌ์์ค๋ฅผ ๋ซ์์ผ ํ๋๋ฐ์, ์ด ๊ธฐ๋ฅ ๋๋ถ์ ์ฝ๋๋ ๊ฐ๊ฒฐํด์ง๊ณ ์ค๋ฅ ๊ฐ๋ฅ์ฑ๋ ์ค์ด๋ญ๋๋ค.
๋ฆฌ์์ค๋ java.lang.AutoCloseable ์ธํฐํ์ด์ค๋ฅผ ๊ตฌํํด์ผ๋ง ์ฌ์ฉํ ์ ์์ต๋๋ค.
๊ธฐ๋ณธ ์ฌ์ฉ๋ฒ
try (ResourceType resource = new ResourceType()) {
// ๋ฆฌ์์ค๋ฅผ ์ฌ์ฉํ๋ ์์
} catch (Exception e) {
// ์์ธ ์ฒ๋ฆฌ
}
์์ ์ฝ๋
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class TryWithResourcesExample {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("example.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
BufferedReader์ FileReader๋ฅผ ์ฌ์ฉํด ํ์ผ์ ์ฝ๋ ์์ ์ ๋๋ค. ๋ฆฌ์์ค๋ ์์ ์ด ๋๋๋ฉด ์๋์ผ๋ก ๋ซํ๋๋ค.
AutoCloseable vs Closeable
๋ฆฌ์์ค๋ฅผ ์ฌ์ฉํ ์ ์๋ ์กฐ๊ฑด์ ๋ ๊ฐ์ง ์ธํฐํ์ด์ค ์ค ํ๋๋ฅผ ๊ตฌํํ๋ ๊ฒ์ ๋๋ค.
- AutoCloseable: Java 7์์ ๋์ ๋ ์ธํฐํ์ด์ค๋ก ๋ชจ๋ ๋ฆฌ์์ค๊ฐ ๊ตฌํํ ์ ์์ต๋๋ค.
- Closeable: I/O ๊ด๋ จ ํด๋์ค์์ ์ฃผ๋ก ์ฌ์ฉ๋๋ฉฐ, AutoCloseable์ ํ์ ์ธํฐํ์ด์ค์ ๋๋ค.
AutoCloseable์ close() ๋ฉ์๋๋ Exception์ ๋์ง ์ ์์ง๋ง, Closeable์ close() ๋ฉ์๋๋ IOException๋ง ๋์ง๋๋ค.
์ฌ๋ฌ ๋ฆฌ์์ค ์ฒ๋ฆฌ
try-with-resources๋ ์ฌ๋ฌ ๋ฆฌ์์ค๋ฅผ ์ธ๋ฏธ์ฝ๋ก (;)์ผ๋ก ๊ตฌ๋ถํ์ฌ ์ฒ๋ฆฌํ ์ ์์ต๋๋ค.
์์
try (
BufferedReader br = new BufferedReader(new FileReader("example.txt"));
FileWriter fw = new FileWriter("output.txt")
) {
String line;
while ((line = br.readLine()) != null) {
fw.write(line + "\n");
}
} catch (IOException e) {
e.printStackTrace();
}
๊ธฐ์กด ๋ฐฉ์๊ณผ ๋น๊ต
์ ํต์ ์ธ try-finally ๋ฐฉ์
BufferedReader br = null;
try {
br = new BufferedReader(new FileReader("example.txt"));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
์ฝ๋๊ฐ ๊ธธ๊ณ ๋ณต์กํ์ฃ ? try-with-resources๋ฅผ ์ฌ์ฉํ๋ฉด ์ด๋ฐ ๋ฒ๊ฑฐ๋ก์์ ์์จ ์ ์์ต๋๋ค.
๋ฆฌ์์ค ๋ซ๊ธฐ ์์
๋ฆฌ์์ค๋ ์ ์ธ๋ ์ญ์์ผ๋ก ๋ซํ๋๋ค.
์์
try (
ResourceA a = new ResourceA();
ResourceB b = new ResourceB()
) {
// ์์
์ํ
}
์ ์ฝ๋์์๋ ResourceB๊ฐ ๋จผ์ ๋ซํ๊ณ , ๊ทธ๋ค์ ResourceA๊ฐ ๋ซํ๋๋ค.
์ปค์คํ ๋ฆฌ์์ค ๋ง๋ค๊ธฐ
์ฌ์ฉ์ ์ ์ ํด๋์ค๋ AutoCloseable ์ธํฐํ์ด์ค๋ฅผ ๊ตฌํํ๋ฉด ์ฌ์ฉํ ์ ์์ต๋๋ค.
์์
class CustomResource implements AutoCloseable {
@Override
public void close() {
System.out.println("๋ฆฌ์์ค๊ฐ ๋ซํ์ต๋๋ค.");
}
}
public class CustomResourceExample {
public static void main(String[] args) {
try (CustomResource resource = new CustomResource()) {
System.out.println("๋ฆฌ์์ค๋ฅผ ์ฌ์ฉ ์ค์
๋๋ค.");
}
}
}
์ถ๋ ฅ ๊ฒฐ๊ณผ:
๋ฆฌ์์ค๋ฅผ ์ฌ์ฉ ์ค์
๋๋ค.
๋ฆฌ์์ค๊ฐ ๋ซํ์ต๋๋ค.
์์ธ ์ฒ๋ฆฌ ๊ฐ์
try-with-resources๋ ๋ฆฌ์์ค๋ฅผ ๋ซ๋ ๊ณผ์ ์์ ๋ฐ์ํ ์์ธ๋ฅผ ์ต์ (suppression)ํ์ฌ ์ฃผ์ ์์ธ์ ํฌํจ์ํต๋๋ค. ์ต์ ๋ ์์ธ๋ getSuppressed() ๋ฉ์๋๋ก ํ์ธํ ์ ์์ต๋๋ค.
์์
public class SuppressedExceptionExample {
public static void main(String[] args) throws Exception {
try (ResourceA a = new ResourceA()) {
throw new Exception("์ฃผ์ ์์ธ");
}
}
}
class ResourceA implements AutoCloseable {
@Override
public void close() throws Exception {
throw new Exception("๋ซ๊ธฐ ์์ธ");
}
}
try-with-resources์ ์ฅ์
- ์ฝ๋ ๊ฐ์ํ: finally ๋ธ๋ก ์์ด ๋ฆฌ์์ค๋ฅผ ๋ซ์ ์ ์์.
- ์์ ์ฑ ํฅ์: ๋ฆฌ์์ค๋ฅผ ์๋์ผ๋ก ๋ซ์ ๋์ ๋ฐฉ์ง.
- ๊ฐ๋ ์ฑ ํฅ์: ๋ช ํํ๊ณ ๊ฐ๊ฒฐํ ์ฝ๋ ์์ฑ ๊ฐ๋ฅ.
- ์์ธ ์ต์ ๊ด๋ฆฌ: ๋ซ๊ธฐ ๊ณผ์ ์์ ๋ฐ์ํ ์์ธ๋ฅผ ์ฒด๊ณ์ ์ผ๋ก ์ฒ๋ฆฌ.
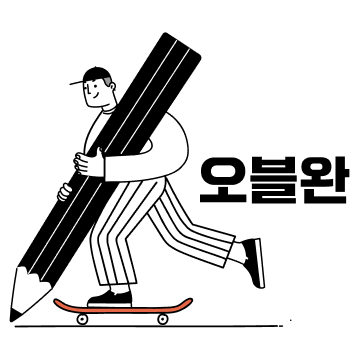
'Back_End > JAVA' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
๋์์ธ ํจํด ์ด๋? + ์์ (0) | 2025.01.03 |
---|---|
์๋ฐ HashMap vs HashTable vs ConcurrentHashMap (1) | 2024.12.31 |
์๋ฐ์์ Generic(์ ๋ค๋ฆญ)์ ์ฐ๋ ์ด์ (1) | 2024.12.27 |
์๋ฐ(Java)์ ๋ฉ๋ชจ๋ฆฌ ์์ญ (1) | 2024.12.19 |
์๋ฐ(JAVA) JRE, JDK, JVM ๋? (1) | 2024.12.18 |